Microservices have become the go-to architectural style for modern software development. They enable teams to build, scale, and deploy applications with greater agility. However, without proper design and management, microservices can introduce complexity. This makes development, collaboration, and deployment more challenging. In this guide, we’ll cover microservices best practices to help you streamline development, improve performance, and maintain system reliability.
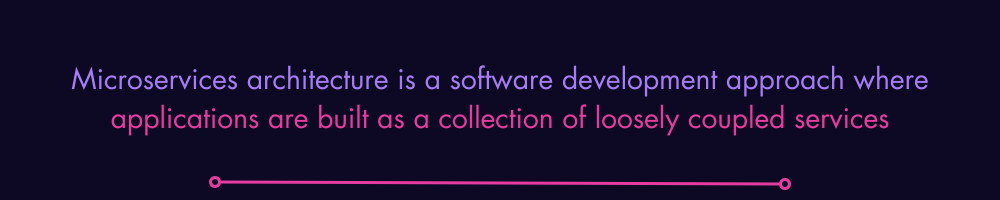
What Are Microservices?
Microservices architecture is a software development approach where applications are built as a collection of loosely coupled services. Each service is independent, performing a specific function, and communicates with other services via APIs. Unlike monolithic applications, microservices enable scalability, flexibility, and faster deployment cycles.
Advantages of Microservices
- Scalability: Each service can be scaled independently, optimizing resource usage. This allows businesses to handle increased workloads efficiently without affecting the entire system.
- Flexibility in Technology Stack: Teams can choose different programming languages, frameworks, and databases for different services, allowing the best technology choices for specific use cases.
- Improved Fault Isolation: Failures in one service do not bring down the entire application, ensuring high availability and reliability.
- Faster Deployments: Individual services can be updated and deployed independently, reducing time to market and enabling continuous delivery.
- Enhanced Maintainability: With services decoupled, teams can iterate and improve individual components without risking unintended impacts on other parts of the system.
Microservices Best Practices
Adopting microservices requires thoughtful design and management to avoid pitfalls. Below are the microservices best practices to ensure success in your development process:
1. Design Microservices with the Single Responsibility Principle (SRP)
The single responsibility principle (SRP) states that each microservice should have only one reason to change. This ensures that each service focuses on a single business capability, making it easier to maintain, test, and scale independently. Violating this principle leads to bloated services that become difficult to manage.
For example, an e-commerce platform should have separate services for order processing, payment handling, and customer management rather than combining all functionalities into a single service.
To implement SRP effectively, do the following:
- Identify distinct business capabilities and map them to separate microservices.
- Keep service responsibilities minimal and focused.
- Avoid tight coupling between services to ensure modularity.
2. Define Clear Service Boundaries
Well-defined service boundaries prevent overlapping responsibilities and ensure smooth communication between services. Service boundaries are typically designed using the bounded context principle from domain-driven design (DDD). Each service should encapsulate its logic and data, reducing dependency on other services.
To establish effective service boundaries, do the following:
- Use domain-driven design (DDD) to define service boundaries based on business logic.
- Keep API contracts clear and concise to minimize integration issues.
- Regularly review and refactor boundaries to accommodate business evolution.
3. Use Independent Databases for Each Service
One of the fundamental principles of microservices architecture is database per service. Sharing databases across services creates dependencies, making it difficult to scale and deploy services independently.
Best practices for managing databases in microservices include:
- Assign each service its dedicated database to ensure data autonomy.
- Use event-driven communication (e.g., Kafka, RabbitMQ) to sync data across services.
- Implement database replication or change data capture (CDC) strategies for consistency.
4. Implement API Gateways for Efficient Communication
An API gateway acts as an entry point for client requests, routing them to the appropriate microservices while handling concerns like authentication, rate limiting, and request transformation.
Benefits of using an API gateway:
- It simplifies client interactions by providing a single access point.
- It enhances security with authentication and authorization mechanisms (e.g., OAuth 2.0, JWT).
- It improves performance by caching responses and load-balancing requests.
Popular API gateway solutions include Kong, NGINX, AWS API Gateway, and Traefik.
5. Ensure Consistent Authentication and Authorization
Security is a critical concern in microservices architecture. A consistent authentication and authorization strategy helps maintain security without adding complexity to individual services.
Recommended authentication strategies to use:
- Use OAuth 2.0 or OpenID Connect (OIDC) for authentication across services.
- Implement JSON Web Tokens (JWT) for stateless authentication.
- Employ role-based access control (RBAC) or attribute-based access control (ABAC) for authorization.
6. Establish Centralized Observability and Monitoring
Observability ensures you can detect, diagnose, and resolve issues across distributed services. A robust observability strategy includes logging, tracing, and metrics collection.
Key observability practices to implement:
- Use centralized logging (e.g., ELK Stack, Fluentd, Loki) to aggregate logs across services.
- Implement distributed tracing (e.g., Jaeger, OpenTelemetry) to track requests across microservices.
- Collect metrics (e.g., Prometheus, Datadog) to monitor performance and detect anomalies.
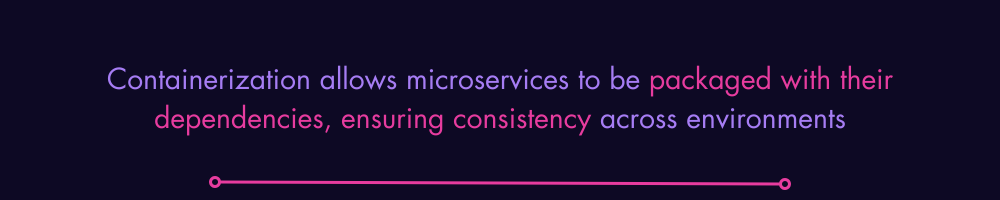
7. Deploy Microservices Using Containerization and Orchestration
Containerization allows microservices to be packaged with their dependencies, ensuring consistency across environments. Kubernetes has become the go-to tool for orchestrating containerized microservices.
Best deployment practices to use:
- Use Docker to package microservices as lightweight, portable containers.
- Employ Kubernetes to automate deployment, scaling, and management.
- Implement service mesh solutions (e.g., Istio, Linkerd) for advanced networking, security, and observability.
8. Adopt CI/CD for Automated Deployment
Continuous integration/continuous deployment (CI/CD) pipelines automate the build, test, and deployment process, ensuring rapid and reliable delivery.
CI/CD best practices to use:
- Use version control systems (e.g., Git) to manage code changes.
- Automate builds and tests using CI tools (e.g., Jenkins, GitHub Actions, GitLab CI/CD).
- Deploy services incrementally using blue-green deployments or canary releases to reduce risk.
9. Optimize Inter-Service Communication
Efficient communication between microservices minimizes latency and enhances performance. Here are communication patterns to consider:
- Synchronous communication: Use REST or gRPC for real-time requests.
- Asynchronous communication: Implement messaging queues (e.g., Kafka, RabbitMQ) for event-driven interactions.
- Circuit breakers: Use tools like Hystrix to handle failures gracefully.
10. Implement Effective Error Handling and Fault Tolerance
Microservices should be designed to handle failures gracefully and ensure high availability. Here are the error-handling strategies to use:
- Use retries with exponential backoff to avoid overwhelming services.
- Implement circuit breakers to prevent cascading failures.
- Log errors centrally and set up alerts using tools like Prometheus Alertmanager or PagerDuty.
11. Choose the Right Deployment Strategy
The best way to deploy microservices depends on the business requirements, system complexity, and desired level of availability. You should consider these approaches when deploying:
- Rolling deployments: Gradually updating services to ensure minimal disruption.
- Blue-green deployments: Running two identical environments to switch traffic seamlessly and eliminate downtime.
- Canary deployments: Deploying new versions to a subset of users before full rollout.
- Serverless deployment: Using platforms like AWS Lambda and Google Cloud Functions for event-driven execution.
12. Optimize for Scalability and Performance
Designing microservices with scalability in mind ensures they can handle varying workloads efficiently. Some key strategies to use are as follows:
- Auto-scaling: Leveraging Kubernetes Horizontal Pod Autoscaler (HPA) or AWS Auto Scaling to scale services dynamically.
- Database partitioning: Using sharding techniques to distribute data loads.
- Load balancing: Deploying services behind a load balancer to distribute requests evenly.
- Caching: Implementing caching mechanisms like Redis or Memcached to reduce database queries and improve response times.
13. Manage Configuration With Externalized Configurations
Storing configurations outside of application code ensures flexibility across environments. You can use tools like:
- Spring Cloud Config for Java-based Microservices
- Consul for service discovery and configuration management
- etcd or Zookeeper for distributed configurations
Frequently Asked Questions
What Are the Three Cs of Microservices?
The three Cs of microservices are:
- Componentization
- Communication
- Coordination
Componentization breaks applications into independent services for scalability and maintainability. Communication ensures seamless data exchange through APIs using protocols like HTTP/REST or gRPC. Coordination manages service discovery, load balancing, and failure recovery to maintain system reliability.

What Is the Best Protocol Between Microservices?
The best protocol depends on the use case. gRPC is preferred for high-performance communication due to its binary serialization, while REST remains popular for simplicity and interoperability. Event-driven architecture using Kafka or RabbitMQ is ideal for asynchronous communication.
Make Your Microservices Architecture Cost-Efficient
Operating microservices at scale in Kubernetes introduces new challenges around resource efficiency and cost management. Without visibility into how workloads consume CPU and memory, teams often overprovision resources to avoid performance issues — resulting in unnecessary cloud spend.
A Kubernetes cost monitoring solution provides insight into resource usage across services, namespaces, and teams. This visibility helps identify overprovisioned workloads and enables data-driven decisions about resource allocation.
With cost optimization in place, compute resources can be right-sized automatically based on actual demand. This reduces waste, maintains reliability, and ensures your Kubernetes infrastructure remains efficient as your architecture grows.